Create a Custom Entity Service Object
Before
we jump into creating a new Entity Service object, it’s worth
discussing what an Entity Service object is and how it relates to the
rest of the items in our model. A BDC model project consists of three
things:
A BDCM file that contains the configuration metadata for the connection
An Entity Service class that contains the methods that will be the endpoints of the operations defined in the metadata
An Entity class that contains the data to be processed by the Entity Service methods
To relate this back to the connection we made
earlier via SharePoint Designer, the BDCM file contains the
configuration details that we entered in the Operations Design View of
the External Content Type. The Entity Service object is the equivalent
of the ADO. NET provider for SQL Server and the Entity class represents
a row of data.
Note
These relationships and their representation in the
design tools can cause some confusion at first glance. In the Entity
View, it appears as though the methods are defined on the entity
object. In fact, the methods are declared as static methods on the
Entity Service object.
Now
that you have a clear understanding of the purpose of the Entity
Service object, we can move on to add the code required to support our
data source.
Since the Bing.com search service is accessed via a web service, we need to add a new service reference. Choose Project | Add Service Reference.
In the Add Service Reference dialog, enter http://api.bing.net/search.wsdl?AppID=<Your App ID Goes Here> &Version=2.2.
Click Go to download the service WSDL.
Set the Namespace to Microsoft.Bing, and then click OK to create proxy classes for the service, as shown here:
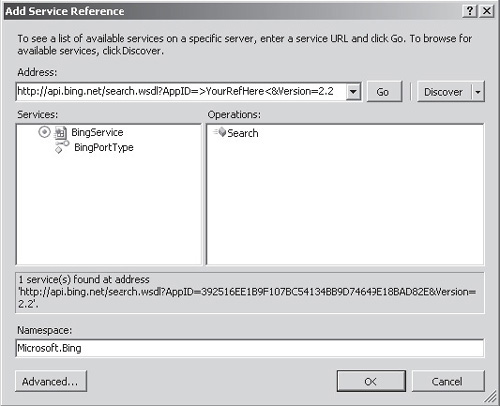
Define a Method to Support the Finder Stereotype
Our next step is to implement methods that will be
called by BCS clients to interact with the data source. In our
demonstration scenario, we need to be able to query the data source
using a wildcard query and retrieve a single specific result.
First of all, let’s take a look at the query method.
You’ll remember from earlier examples that the operation for retrieving
a list of data is referenced using the Finder stereotype. We’ll start
by creating a new method for this stereotype.
In
the Entity viewer in Visual Studio, delete the default Entity1 object,
and then drag a new Entity from the toolbox onto the design surface.
Change the Entity name to WebResult.
In
the BDC Methods Details pane, click <Add a Method> to create a
new method. From the drop-down that appears, select Create Finder
Method. This will automatically add a method named ReadList.
Since
our Finder method needs to be able to perform wildcard searches, we
need to add a wildcard filter. We’re effectively performing the same
configuration that we did earlier when we set up a search filter on the
Model External Content Type—you’ll no doubt recognize some of the
configuration objects that we’re dealing with. To add a new filter, we
first need a parameter to which we can attach the filter. We’ll add a
new query parameter to the ReadList
method. Expand the parameters node in the BDC Method Details pane, and
then click Add a Parameter. Select Create A Parameter to add a new
parameter to the method. You’ll notice that although the default
parameter name is selected in the BDC Method Details pane, it’s not
possible to overtype it with a new value. To add a new value, we need
to use the Properties pane, as shown next. Type query for the new parameter name.
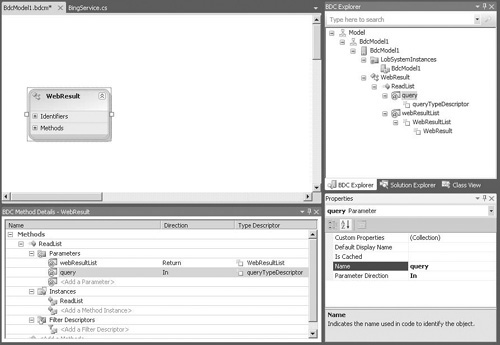
With
this new parameter in place, we’re now free to add a new filter. Under
the Filter Descriptors node, click <Add a Filter Descriptor>, and
then select Create a Filter Descriptor. As before, use the Properties
pane to change the Name to Search Filter and the Type to Wildcard.
To make this filter the default, we need to add an additional property.
Click the ellipsis next to Custom Properties in the Properties pane to
add another property. Type IsDefault for the Name, System.Boolean for the Type, and true for the Value.
Now that we have a filter, we need to associate it with our ReadList
method. We can do this by selecting an associated type description.
You’ll notice in the BDC Method Details pane that each parameter has a
type descriptor attached. A type descriptor is metadata
that defines the type of the parameter as well as a number of
properties that control how the type should be handled, such as whether
the type is an identifier or should be read-only. When we added our
additional parameter to the ReadList
method, a new type descriptor was automatically created. We need to
attach our Search Filter to this type. From the BDC Explorer pane,
expand the ReadList node and the query node beneath it. Select the
queryTypeDescriptor node to define the properties of the parameter.
First, let’s change the name to something more succinct: In the
Properties pane, change the Name to simply query.
To attach the filter to this type, select the filter name (Search
Filter) from the drop-down list next to the Associated Filter property,
as illustrated next:
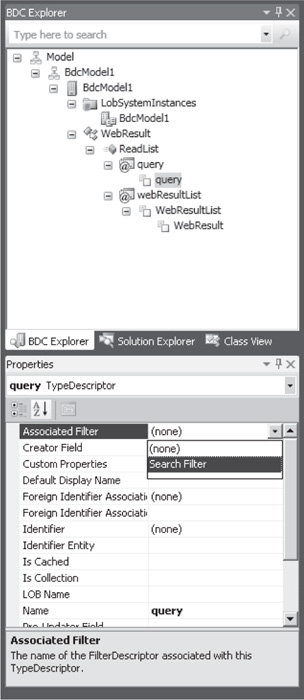