5. Flexible Database Roles
If your database consisted of just a couple of
users, it would be very easy for you to manage permission for these
users directly. However, in the real world, DBAs manage lots of users
and, more commonly, many different types of users. A developer will
have different requirements than a business analyst. If your
organization has 35 developers and 70 business analysts, you have a lot
of permissions to manage. To alleviate this burden, you can create a
database role, add database users or other roles to this new role, and
assign permissions to the role. Now, any time you have new developers,
all you have to do is add their usernames to the role, and they have
all the necessary permissions.
To create a database role, use the CREATE ROLE
statement, as shown here:
USE AdventureWorks
GO
CREATE ROLE Developers AUTHORIZATION DevManager
GO
Here, you are creating a new role, Developers
, and making the DevManager
user the owner of this new role. As an owner, you can freely add and remove membership to the role.
To add users to the role, use the sp_addrolemember
stored procedure as follows:
sp_addrolemember 'Developers', 'Bryan'
This assumes that there is a database user within the database named Bryan.
Once you defined a role, you can grant permission to the role using the GRANT
statement as follows:
GRANT CREATE TABLE TO Developers
Note Even though DevManager
may be the owner of the Developers
role, DevManager
would still need the ability to grant the CREATE TABLE
permission in order for the previous statement to work. To do this, the DBA would issue the GRANT
statement with the WITH GRANT OPTION
clause as follows:
GRANT CREATE TABLE TO DevManager WITH GRANT OPTION
6. Security Functions
Now that you know how to grant, revoke, and
deny permissions, I’ll introduce a series of functions that are
designed to help you in managing security. Although most of this
information is available in SQL Server Management Studio dialog boxes,
the following functions and catalog views are useful if you prefer to
issue Transact-SQL statements instead of using the UI.
fn_my_permissions() Function
You also granted CREATE TABLE
permission to this role. If Bryan wanted to know what permissions he
had within this database, he could use SQL Server Management Studio or
simply leverage the fn_my_permissions
function as follows:
SELECT * FROM fn_my_permissions(NULL, 'DATABASE');
For Bryan, this query would return the information in Table 3.
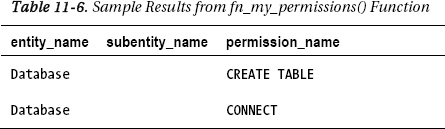
This function also works at the server instance level. By replacing the DATABASE
word with SEVER
, the function will return the server-level permissions that are granted to the login.
HAS_PERMS_BY_NAME Function
You also learned that users can be members of roles, and these roles
can be members of other roles. In the end, if you wanted to really know
what permissions a user had on an object, it would be difficult to
trace through all these layers of indirection. The function tells you
whether the current context has a specific permission. For example, if
developer Bryan wanted to know whether he had SELCT
permission on the Customers
table, he could issue the following query:
SELECT HAS_PERMS_BY_NAME('Customers', 'OBJECT', 'SELECT')
This function will return a 1 or 0, indicating a true or false value, respectively.
If you wanted to know whether another user had a specific permission, you would have to be a sysadmin or have IMPERSONATE
permission for the user in question. Provided one of those conditions are satisfied, you could find out whether Bryan has the SELECT
permission by issuing the following:
EXECUTE AS USER=’Bryan’
GO
SELECT HAS_PERMS_BY_NAME('Customers', 'OBJECT', 'SELECT')
GO