GPS stands for Global Positioning System. GPS is a space-based
satellite navigation system that sends reliable location information to
your handheld device.
If your application requires the use of the device’s GPS
capabilities, you will need to select the read_geolocation permission when creating your
project.
Let’s review the code that follows. First, you’ll notice that there
is a private variable named geoLocation declared of
type flash.sensors.GeoLocation. Within
applicationComplete of the application, an event
handler function is called; it first checks to see if the device has an
available GPS unit by reading the static property of the
GeoLocation class. If this property returns as true, a
new instance of GeoLocation is created and the data refresh interval is set to 500 milliseconds (.5
seconds) within the setRequestedUpdateInterval method, and an
event listener of type GeoLocationEvent.UPDATE is added to
handle updates. Upon update, the GPS information is read from the event
and written to a TextArea within the
handleUpdate function. Note that there is also some
math being done to convert the speed property into miles per hour and
kilometers per hour. The results can be seen within Figure 1.
<?xml version="1.0" encoding="utf-8"?>
<s:Application xmlns:fx="http://ns.adobe.com/mxml/2009"
xmlns:s="library://ns.adobe.com/flex/spark"
applicationComplete="application1_applicationCompleteHandler(event)">
<fx:Script>
<![CDATA[
import mx.events.FlexEvent;
import flash.sensors.Geolocation;
private var geoLocation:Geolocation;
protected function application1_applicationCompleteHandler
(event:FlexEvent):void {
if(Geolocation.isSupported==true){
geoLocation = new Geolocation();
geoLocation.setRequestedUpdateInterval(500);
geoLocation.addEventListener(GeolocationEvent.UPDATE,
handleLocationRequest);
} else {
status.text = "Geolocation feature not supported";
}
}
private function handleLocationRequest(event:GeolocationEvent):void {
var mph:Number = event.speed*2.23693629;
var kph:Number = event.speed*3.6;
info.text = "Updated: " + new Date().toTimeString() + "\n\n"
+ "latitude: " + event.latitude.toString() + "\n"
+ "longitude: " + event.longitude.toString() + "\n"
+ "altitude: " + event.altitude.toString() + "\n"
+ "speed: " + event.speed.toString() + "\n"
+ "speed: " + mph.toString() + " MPH \n"
+ "speed: " + kph.toString() + " KPH \n"
+ "heading: " + event.heading.toString() + "\n"
+ "horizontal accuracy: "
+ event.horizontalAccuracy.toString() + "\n"
+ "vertical accuracy: "
+ event.verticalAccuracy.toString();
}
]]>
</fx:Script>
<fx:Declarations>
<!-- Place non-visual elements (e.g., services, value objects) here -->
</fx:Declarations>
<s:Label id="status" text="Geolocation Info" top="10" width="100%"
textAlign="center"/>
<s:TextArea id="info" width="100%" top="40" editable="false"/>
</s:Application>
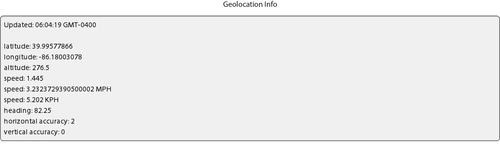