Enabling New Dimensions in Forms
You can enable
new dimensions by setting up dimension groups, but you won’t see them
yet in the forms. The inventory dimension feature uses a temporary table
named InventDimParm to carry certain information, such as whether a dimension has the following attributes:
Is enabled
Is an item dimension
Is a primary stocking dimension
Is visible
Serves as a filter-by term
Serves as a group-by term
Serves as an order-by term
The dimension groups are enabled and controlled by reflecting each inventory dimension as a boolean flag field on the InventDimParm table and then matching the corresponding fields in the X++ code. For example, when a dimension group is queried to determine which dimensions are active, an InventDimParm
record is returned where the corresponding flag field is set to true
for the active dimensions. The remaining flags are set to false. You
must therefore add a frame-size flag and a wheel-size flag to the InventDimParm table, as shown in Table 3.
Table 3. BikeFrameSizeFlag and BikeWheelSizeFlag Property Settings
Property | BikeFrameSizeFlag | BikeWheelSizeFlag |
---|
Type | enum | enum |
Label | Frame size | Wheel size |
HelpText | View by frame size | View by wheel size |
ExtendedDataType | NoYesId | NoYesId |
Enum | NoYes | NoYes |
You should also add the new fields to the FixedView and View field groups defined on the InventDimParm table, because they are used in forms from which it is possible to specify whether a dimension should be visible.
When you add fields to the table and field groups, you must map the new fields on the InventDim table to the corresponding fields on the InventDimParm table in the X++ code. To do this, you modify the dim2DimParm method on the InventDim table, as shown in the following X++ code. The added mappings of BikeFrameSize and BikeWheelSize appear in bold.
static public fieldId dim2dimParm(fieldId dimField) { ; #InventDimDevelop
switch (dimField) { case (fieldnum(InventDim,ConfigId)) : return fieldnum(InventDimParm,ConfigIdFlag); case (fieldnum(InventDim,InventSizeId)) : return fieldnum(InventDimParm,InventSizeIdFlag); case (fieldnum(InventDim,InventColorId)) : return fieldnum(InventDimParm,InventColorIdFlag); case (fieldnum(InventDim,InventSiteId)) : return fieldnum(InventDimParm,InventSiteIdFlag); case (fieldnum(InventDim,InventLocationId)) : return fieldnum(InventDimParm,InventLocationIdFlag); case (fieldnum(InventDim,InventBatchId)) : return fieldnum(InventDimParm,InventBatchIdFlag); case (fieldnum(InventDim,wMSLocationId)) : return fieldnum(InventDimParm,WMSLocationIdFlag); case (fieldnum(InventDim,wMSPalletId)) : return fieldnum(InventDimParm,WMSPalletIdFlag); case (fieldnum(InventDim,InventSerialId)) : return fieldnum(InventDimParm,InventSerialIdFlag); case (fieldnum(InventDim,BikeFrameSize)) : // Add mapping
return fieldnum(InventDimParm,BikeFrameSizeFlag); case (fieldnum(InventDim,BikeWheelSize)) : // Add mapping return fieldnum(InventDimParm,BikeWheelSizeFlag);
}
throw error(strfmt("@SYS54431",funcname())); }
|
You must make the same modification to the dimParm2Dim method on the same table to map InventDimParm fields to InventDim fields.
Customizing Other Tables
The customizations made
so far allow the new dimensions to be enabled on dimension groups and
presented in forms. However, you should also consider customizing the
following tables by adding inventory dimensions to them:
Whether and how you
should customize these tables depends on the functionality you’re
implementing. Be sure to examine how the inventory dimensions are
implemented and used for each of the tables before you begin
customizing.
Adding Dimensions to Queries
Because of the generic implementation of the inventory dimension concept using the InventDim and InventDim Parm
tables, a substantial number of queries written in X++ use just a few
patterns to select, join, and filter the inventory dimensions. So that
you don’t have to repeatedly copy and paste the same X++ code, these
patterns exist as macros that you can
apply in your code. To modify these queries, you simply customize the
macros and then recompile the entire application to update the X++ code
with the new dimensions.
You should customize the following macros:
InventDimExistsJoin
InventDimGroupAllFields
InventDimJoin
InventDimSelect
The bold text in the following X++ code shows the changes that you must make to the InventDimExistsJoin macro to enable the two new dimensions for all exists joins written as statements involving the InventDim table.
/* %1 InventDimId */ /* %2 InventDim */ /* %3 InventDimCriteria */ /* %4 InventDimParm */ /* %5 Index hint */
exists join tableId from %2 where (%2.InventDimId == %1) && (%2.ConfigId == %3.ConfigId || ! %4.ConfigIdFlag) && (%2.InventSizeId == %3.InventSizeId || ! %4.InventSizeIdFlag) && (%2.InventColorId == %3.InventColorId || ! %4.InventColorIdFlag) && (%2.BikeFrameSize == %3.BikeFrameSize || ! %4.BikeFrameSizeFlag) &&
(%2.BikeWheelSize == %3.BikeWheelSize || ! %4.BikeWheelSizeFlag) &&
(%2.InventSiteId == %3.InventSiteId || ! %4.InventSiteIdFlag) && (%2.InventLocationId == %3.InventLocationId || ! %4.InventLocationIdFlag) && (%2.InventBatchId == %3.InventBatchId || ! %4.InventBatchIdFlag) && (%2.WMSLocationId == %3.WMSLocationId || ! %4.WMSLocationIdFlag) && (%2.WMSPalletId == %3.WMSPalletId || ! %4.WMSPalletIdFlag) && (%2.InventSerialId == %3.InventSerialId || ! %4.InventSerialIdFlag)
#InventDimDevelop
|
The three remaining macros
are just as easy to modify. Just remember to recompile the entire
application after you make your changes.
Adding Lookup, Validation, and Defaulting X++ Code
In addition to macro customizations and the customizations to the previously mentioned methods on the InventDim table, you must implement and customize lookup, validation, and defaulting methods. These include methods such as the InventDim::findDim lookup method, the InventDim.validateWriteItemDim validation method, and the InventDim.initFromInventDimCombination defaulting method. The necessary changes in the InventDim::findDim lookup method for the new inventory dimensions are shown in bold in the following X++ code.
server static public InventDim findDim(InventDim _inventDim, boolean _forupdate = false) { InventDim inventDim; ; if (_forupdate) inventDim.selectForUpdate(_forupdate);
select firstonly inventDim where inventDim.ConfigId == _inventDim.ConfigId && inventDim.InventSizeId == _inventDim.InventSizeId && inventDim.InventColorId == _inventDim.InventColorId && inventDim.BikeFrameSize == _inventDim.BikeFrameSize
&& inventDim.BikeWheelSize == _inventDim.BikeWheelSize
&& inventDim.InventSiteId == _inventDim.InventSiteId && inventDim.InventLocationId == _inventDim.InventLocationId && inventDim.InventBatchId == _inventDim.InventBatchId && inventDim.wmsLocationId == _inventDim.wmsLocationId && inventDim.wmsPalletId == _inventDim.wmsPalletId && inventDim.InventSerialId == _inventDim.InventSerialId;
#inventDimDevelop
return inventDim; }
|
Notice the use of the inventDimDevelop macro in the preceding method. This macro contains only the following comment:
/* used to locate code with direct dimension references */
|
Performing a global search for use of the inventDimDevelop
macro should be sufficient to find all the X++ code that you must
consider when implementing a new dimension. This search returns all the
methods that require further investigation. Figure 7 shows results of a search for the use of the macro on all tables.
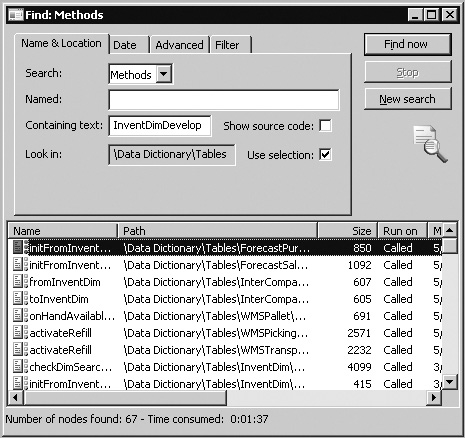
Most of the methods
you find when searching for the macro are lookup, validation, and
defaulting methods, but you also see methods that aren’t in these
categories. Such methods include those that modify the Query object, such as the InventDim::queryAddHintFromCaller method, and methods that describe dimensions, such as InventDimParm.isFlagSelective. You should also review these methods when investigating the X++ code.
Tip
Although the inventory dimension feature is implemented with the inventDimDevelop
macro to direct developers to the methods they need to change, you
might encounter methods with no macro included or tables, forms, or
reports for which the inventory dimensions are not used generically. We
therefore advise you to use the cross-reference system on an existing
dimension that has the same behavior as the new dimension to determine
its use and review it appropriately. You should also investigate whether
the new dimension is or should be available in the same element. |