ViewGroups are Views that contain child Views. Each
ViewGroup class embodies a different set of assumptions
about how to display its child Views. All ViewGroups descend from the
android.view.ViewGroup class. Layouts.
1. Gallery and GridView
The Gallery ViewGroup (Figure 1) displays multiple
items in a horizontally scrolling list. The currently selected item is
locked in the center of the screen. Any items that approach the edge of
the screen begin to fade, giving the user the impression that there may
be more items “around the corner.” The user can scroll horizontally
through the items within the gallery. This ViewGroup is useful when you
want to present a large set of possible choices to the user without
using too much screen real estate.
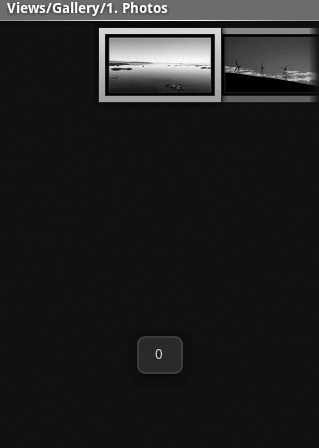
A GridView (Figure 2, shown later) is very
similar to a Gallery. Like a Gallery, the GridView displays many child
Views that the user can manipulate. But in contrast to a Gallery, which
is a one-dimensional list that the user can scroll horizontally, a
GridView is a two-dimensional array that the user can scroll
vertically.
The Gallery and GridView
classes both descend from the AdapterView class, so
you need a subclass of Adapter to provide a standardized way to access
the underlying data. Any class that implements the Adapter class must implement the following
abstract functions from that class:
int getCount
Returns the number of items in the data set represented by
the Adapter.
Object getItem(int
position)
Returns the object in the Adapter function (Adapter
class) at the given position.
long getItem(int
position)
Returns the row ID within the Adapter of the object at the given
position.
View getView(int position, View
convertView, ViewGroup parent)
Returns a View object that will display the data in the
given position in the data set.
The ApiDemos application’s views.Gallery1.java file shows off the
Gallery ViewGroup nicely. The demo displays a variety of images for the
user to select, and when the user does select one, the image’s index
number briefly appears as toast.
The ApiDemos application also includes two example GridView
Activities that show how to use the GridView. We will not examine the
GridView here, because the Gallery example is so similar.
Example 1 shows how to
use a Gallery ViewGroup. Example 1 shows the XML layout
file (gallery_1.xml).
Example 1. Layout file for Gallery example
<?xml version="1.0" encoding="utf-8"?> <Gallery xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/gallery" android:layout_width="fill_parent" android:layout_height="wrap_content" /> |
Here are some of the highlights of the layout code:
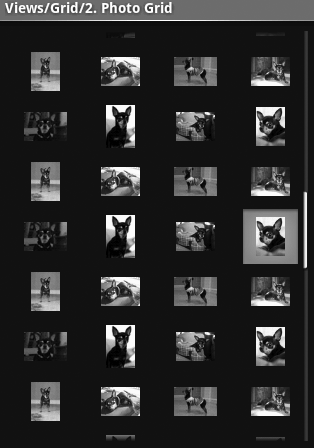
Now we’ll turn our attention to the Java implementation, Gallery1.java, shown in Example 2. We’ve modified the code
from ApiDemos slightly to remove some features that do not add to our
understanding of the Gallery ViewGroup.
Example 2. Java for Gallery: Gallery1.java
public class Gallery1 extends Activity {
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.gallery_1);
// Reference the Gallery view Gallery g = (Gallery) findViewById(R.id.gallery); // Set the adapter to our custom adapter (below) g.setAdapter(new ImageAdapter(this));
// Set a item click listener, and just Toast the clicked position g.setOnItemClickListener(new OnItemClickListener() { public void onItemClick(AdapterView parent, View v, int position, long id) { Toast.makeText(Gallery1.this, "" + position, Toast.LENGTH_SHORT).show(); } }); }
|
Here are some of the highlights of the code:
In Example 2, the
setAdapter function tells the
Gallery object to use the ImageAdapter object as its Adapter. Example 3 defines our ImageAdapter class. This
ImageAdapter implements all of the abstract functions
required in its base class, BaseAdapter. For the
simple case of this demo, picture resources represent the data that the
Gallery view is displaying. An integer array, mImageIds, contains the resource IDs of the
picture resources.
Example 3. Java for Gallery’s Adapter
public class ImageAdapter extends BaseAdapter { int mGalleryItemBackground;
private Context mContext;
private Integer[] mImageIds = { R.drawable.gallery_photo_1, R.drawable.gallery_photo_2, R.drawable.gallery_photo_3, R.drawable.gallery_photo_4, R.drawable.gallery_photo_5, R.drawable.gallery_photo_6, R.drawable.gallery_photo_7, R.drawable.gallery_photo_8 };
public ImageAdapter(Context c) { mContext = c;
TypedArray a = obtainStyledAttributes(android.R.styleable.Theme); mGalleryItemBackground = a.getResourceId( android.R.styleable.Theme_galleryItemBackground, 0); a.recycle(); }
public int getCount() { return mImageIds.length; }
public Object getItem(int position) { return position; }
public long getItemId(int position) { return position; }
public View getView(int position, View convertView, ViewGroup parent) { ImageView i = new ImageView(mContext);
i.setImageResource(mImageIds[position]); i.setScaleType(ImageView.ScaleType.FIT_XY); i.setLayoutParams(new Gallery.LayoutParams(136, 88));
// The preferred Gallery item background i.setBackgroundResource(mGalleryItemBackground);
return i; } } }
|