When we include a speed (or, more precisely, a duration) with .show() or .hide(), it becomes animated—occurring over a specified period of time. The .hide('speed')
method, for example, decreases an element's height, width, and opacity
simultaneously until all three reach zero, at which point the CSS rule display:none is applied. The .show('speed')
method will increase the element's height from top to bottom, width
from left to right, and opacity from 0 to 1 until its contents are
completely visible.
Speeding in
With any jQuery effect, we can use one of three preset speeds:'slow', 'normal', and'fast'. Using .show('slow') makes the show effect complete in .6 seconds, .show('normal') in .4 seconds, and .show('fast') in .2 seconds. For even greater precision we can specify a number of milliseconds, for example .show(850). Unlike the speed names, the numbers are not wrapped in quotation marks.
Let's include a speed in our example when showing the second paragraph of Lincoln's Gettysburg Address:
$(document).ready(function() {
$('p:eq(1)').hide();
$('a.more').click(function() {
$('p:eq(1)').show('slow');
$(this).hide();
return false;
});
});
When we capture the paragraph's appearance at roughly halfway through the effect, we see something like the following:
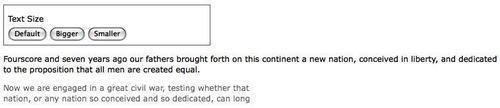
Fading in and fading out
While the animated .show() and .hide()
methods are certainly flashy, they may at times be too much of a good
thing. Fortunately, jQuery offers a couple other pre-built animations
for a more subtle effect. For example, to have the whole paragraph
appear just by gradually increasing the opacity, we can use .fadeIn('slow') instead:
$(document).ready(function() {
fadeIn(slow) methodusing$('p:eq(1)').hide();
$('a.more').click(function() {
$('p:eq(1)').fadeIn('slow');
$(this).hide();
return false;
});
});
This time when we capture the paragraph's appearance halfway, it's seen as:
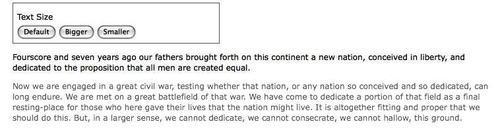
The difference here is that the .fadeIn()
effect starts by setting the dimensions of the paragraph so that the
contents can simply fade into it. To gradually decrease the opacity we
can use .fadeOut().