A camera is available on all BlackBerry Tablet devices.
If your application requires the use of the device’s camera, you
will need to select the use_camera
permission to access the camera and the access_shared permission to read the new image
when you’re creating your project.
Let’s review the following code. First, you'll notice that there is
a private variable named camera declared of type
flash.media.CameraUI. Within
applicationComplete of the application, an event
handler function is called; it first checks to see if the device has an
available Camera by reading the static property of the
CameraUI class. If this property returns as true, a new instance of
CameraUI is created and event listeners of type
MediaEvent.COMPLETE and
ErrorEvent.COMPLETE are added to handle
a successfully captured image, as well as any errors that may
occur.
A Button with an event listener on the click
event is used to allow the application user
to launch the CameraUI. When the user clicks
the TAKE A PICTURE button, the captureImage method is
called, which then opens the camera by calling the
launch method and passing in the MediaType.IMAGE static property. At this point,
the user is redirected from your application to the native camera. Once
the user takes a picture and clicks OK, he is directed back to your
application, the MediaEvent.COMPLETE
event is triggered, and the onComplete method is
called. Within the onComplete method, the event.data property is cast to a
flash.Media.MediaPromise object. The
mediaPromise.file.url property is then used to
populate Label and Image components
that display the path to the image and the actual image to the
user.
Note:
Utilizing CameraUI within your application is
different than the raw camera access provided by Adobe AIR on the
desktop. Raw camera access is also available within AIR on BlackBerry
Tablet OS and works the same as the desktop version.
Figure 1 shows the application,
Figure 2 shows the native camera user
interface, and Figure 3 shows
the application after a picture is taken and the user clicks OK to return
to the application.
<?xml version="1.0" encoding="utf-8"?>
<s:Application xmlns:fx="http://ns.adobe.com/mxml/2009"
xmlns:s="library://ns.adobe.com/flex/spark"
applicationComplete="application1_applicationCompleteHandler(event)">
<fx:Script>
<![CDATA[
import mx.events.FlexEvent;
private var camera:CameraUI;
protected function application1_applicationCompleteHandler
(event:FlexEvent):void {
if (CameraUI.isSupported){
camera = new CameraUI();
camera.addEventListener(MediaEvent.COMPLETE, onComplete);
camera.addEventListener(ErrorEvent.ERROR, onError);
status.text="CameraUI supported";
} else {
status.text="CameraUI NOT asuported";
}
}
private function captureImage(event:MouseEvent):void {
camera.launch(MediaType.IMAGE);
}
private function onError(event:ErrorEvent):void {
trace("error has occurred");
}
private function onComplete(event:MediaEvent):void {
var mediaPromise:MediaPromise = event.data;
status.text = mediaPromise.file.url;
image.source = mediaPromise.file.url;
}
]]>
</fx:Script>
<fx:Declarations>
<!-- Place non-visual elements (e.g., services, value objects) here -->
</fx:Declarations>
<s:Label id="status" text="Click Take a Picture button" top="10" width="100%"
textAlign="center"/>
<s:Button width="300" height="60" label="TAKE A PICTURE"
click="captureImage(event)"
horizontalCenter="0" enabled="{CameraUI.isSupported}"
top="80"/>
<s:Image id="image" width="230" height="350" horizontalCenter="0" top="170"/>
</s:Application>
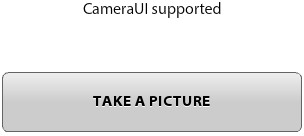
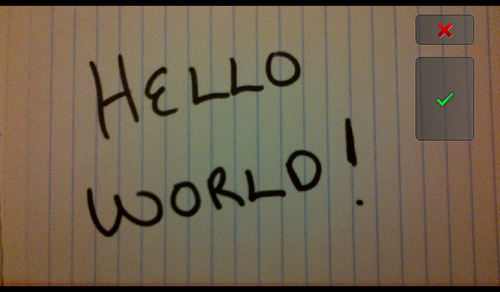
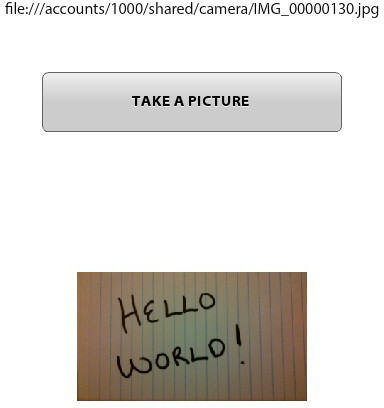