Although we couldn’t use LINQ to add document items
to a document library, we can use LINQ to delete such items. Delete
works because LINQ provides a DeleteOnSubmit method that makes use of
the unique identifier for the entity object to delete the corresponding
object from the data store.
1. Deleting Sample Data
Add a new button to the sample application and name it Delete Sample Data.
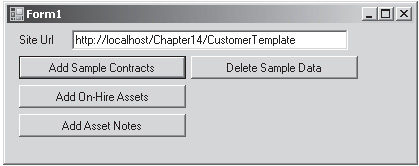
Add the following code in the on-click event handler:
private void button4_Click(object sender, EventArgs e)
{
//disable the button to prevent concurrency problems
button4.Enabled = false;
using(HireSampleDataContext dxWrite = new HireSampleDataContext(SiteUrl.Text))
{
dxWrite.ObjectTrackingEnabled = true;
//loop through the Hire Contracts
foreach (HireContract contract in dxWrite.HireContracts)
{
//for each contract, loop through the assets
foreach (OnHireAsset asset in contract.OnHireAsset)
{
//for each asset loop through the notes
foreach (AssetNote note in asset.AssetNote)
{
//delete the note
dxWrite.AssetNotes.DeleteOnSubmit(note);
}
//delete the asset
dxWrite.OnHireAssets.DeleteOnSubmit(asset);
}
//finally delete the contract
dxWrite.HireContracts.DeleteOnSubmit(contract);
}
dxWrite.SubmitChanges();
}
//change the text on the button so that we know the job's done
button4.Text += " - Done";
}
It should be apparent from the comments that this
code will delete all the sample data that we created in the preceding
code examples. It uses three nested loops to ensure that referential
integrity is maintained, and all associated child data is deleted
before the parent element is deleted.