2. Button and ImageButton
The Button View is just a button, printed with some text to identify
it, that the user can click to invoke some action. The previous section
created a Button and connected it to an OnClickListener
method that executes when the Button is clicked.
Android has a very visual, mobile-oriented user interface, so you
might want to use a button with an image on it rather than one with
text. Android provides the ImageButton View for just that purpose. You
can adapt Example 2 to use an
ImageButton by making one change in the XML file and another in the Java
code:
In main.xml, replace the
Button definition for btnDone
with an ImageButton:
...
<ImageButton
android:id="@+id/btnDone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
...
In TextViewDemo.java,
redefine btn1 as an ImageButton
and add a line to set the image to a PNG image in the drawable directory:
...
private static ImageButton btn1;
...
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//Get pointers to the Views defined in main.xml
txt1 = (TextView) findViewById(R.id.txtDemo);
etxt1 = (EditText) findViewById(R.id.eTxtDemo);
btn1 = (ImageButton) findViewById(R.id.btnDone);
...
//Set the image for the Done button
btn1.setImageResource(R.drawable.log);
...
The button now appears as shown in Figure 2.
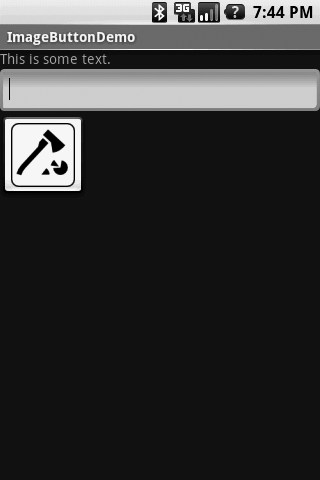
3. Adapters and AdapterViews
The AdapterView is a generic, list-oriented
view of data. Any collection of data objects that can be ordered in some
relatively stable way can be displayed through an AdapterView. An
AdapterView is always associated with an
Adapter, which acts as the bridge between it and the
underlying data collection. The Adapter has two
responsibilities:
At the request of the AdapterView, the
Adapter must be able to find the data object that
corresponds to a particular index. It must, in other words, be able
to find the data object that is visible in the
AdapterView at a particular location.
Inversely, the Adapter must be able to
supply a view through which the data at a particular index can be
displayed.
It takes only a moment’s reflection to understand how the
AdapterView works: It is a
ViewGroup that contains all the machinery necessary
to serve as both the View and Controller for a collection of generic
widgets. It can lay them out on the display, pass in clicks and
keystrokes, and so on. It need never concern itself with what the
subviews actually display; it distinguishes them only by their indexes.
Whenever it needs to perform either of the two operations that are not
entirely generic—creating a new view or getting the data object attached
to a particular view—it relies on the Adapter to
convert an index into either a data object or the view of a data
object.
The AdapterView requests new views from an
implementation of the Adapter interface, as it needs
them, for display. For instance, as a user scrolls though a list of
contacts, the AdapterView requests a new view for
each new contact that becomes visible. As an optimization, the
AdapterView may offer a view that is no longer
visible (in this case, one that has scrolled off the display) for reuse.
This can dramatically reduce memory churn and speed up display.
When offered a recycled view, however, the
Adapter must verify that it is the right kind of view
through which to display the data object at the requested index. This is
necessary because the Adapter is not limited to
returning instances of a single view class in response to the request
for a view. If the Adapter represents several kinds
of objects, it might create several different types of views, each
applicable to some subset of the data objects in the collection. A list
of contacts, for instance, might have two entirely different view
classes: one for displaying acquaintances that are currently online and
another for those who are not. The latter might completely ignore
clicks, whereas the former would open a new chat session when
clicked.
Although AdapterView and
Adapter are both abstract and cannot be directly
instantiated, the UI toolkit includes several prebuilt
Adapters and AdapterViews that can
be used unmodified or further subclassed to provide your own
customizations. ListAdapter and SpinnerAdapter are
particularly useful Adapters, while
ListView, GridView, Spinner, and
Gallery are all handy subclasses of
AdapterView. If you plan to create your own subclass
of AdapterView, a quick look at the code for one of
these classes will get you off to a running start.