Lots of the graphics in games are faked. Rather than
make something 3-D, a game programmer makes something that looks 3-D but
turns out to be much easier to program. In this section, you make some
3-D text, but without using any complicated rendering or models
(although you can do this kind of thing if required). You use only two
principles:
This means that you need to
draw your text in three stages. First, you draw the shadows, then the
"sides" of the text, and finally the top layer of the text. This seems
like a lot of work, but, as Figure 1 shows, I think it’s worth the effort.
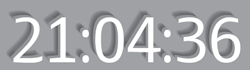
1. Creating Shadows Using Transparent Colors
The first part of the text
that you want to draw is the shadow at the back. You draw your picture
from the back forwards and use the fact that each time you draw, you add
to what’s already there. You use another feature of XNA drawing: colors
that cause things to be drawn slightly transparent (that is, with part
of the background showing through). By drawing transparent colors on top of each other, you can get a nice blurry effect, as done in the following code:
Color nowColor = new Color(0,0,0,20);
for (layer = 0; layer < 10 ; layer++)
{
spriteBatch.DrawString(font, nowString, nowVector, nowColor);
nowVector.X++;
nowVector.Y++;
}
This code is very similar to the previous code that draws the 3-D text except that it creates the value for nowColor in a slightly different way. The Color is constructed from four values rather than three:
Color nowColor = new Color(0,0,0,20);
The first three values give
the intensity of red, green, and blue, which you’ve set to 0 because
you’re drawing in black. The fourth gives the transparency of the color.
In graphical terms, this is often called the alpha
channel value. The bigger the number, the less the background shows
through. Just like your color intensity values, the transparency value
can range from 0 (completely transparent) to 255 (solid color). If you
don’t give a transparency value, the Color is created as solid.
A value of 20 means that a lot of the background shows through the color that you draw. Figure 2
shows the display produced by drawing 10 times using a transparent
black value. Note that because each of the drawing positions is slightly
different, you get a blurring effect.
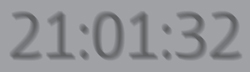
This works rather well in
that the text is nicely blurred around the edges, as a shadow would be.
Now you know one way video games achieve blur. They can do it by
repeatedly drawing the same scene in slightly different positions.
The next part of the
drawing process uses the same technique you’ve used before, except that
you use slightly different colors. The complete drawing method is as
follows:
protected override void Draw(GameTime gameTime)
{
graphics.GraphicsDevice.Clear(Color.CornflowerBlue);
DateTime nowDateTime = DateTime.Now;
string nowString = nowDateTime.ToLongTimeString();
Vector2 nowVector = new Vector2(50, 400);
int layer;
spriteBatch.Begin();
// Draw the shadow
Color nowColor = new Color(0, 0, 0, 20);
for (layer = 0; layer < 10; layer++)
{
spriteBatch.DrawString(font, nowString, nowVector, nowColor);
nowVector.X++;
nowVector.Y++;
}
// Draw the solid part of the characters
nowColor = Color.Gray;
for (layer = 0; layer < 5; layer++)
{
spriteBatch.DrawString(font, nowString, nowVector, nowColor);
nowVector.X++;
nowVector.Y++;
}
// Draw the top of the characters
spriteBatch.DrawString(font, nowString, nowVector, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
This produces the display shown in Figure 2.
2. Drawing Images with Transparency
Something else that’s
useful is that if you draw an image using a color that has a
transparency value, the image is drawn transparently. This is how game
programmers get pictures to fade slowly onto the screen. The image is
repeatedly drawn with different levels of transparency to make it slowly
appear over a background.